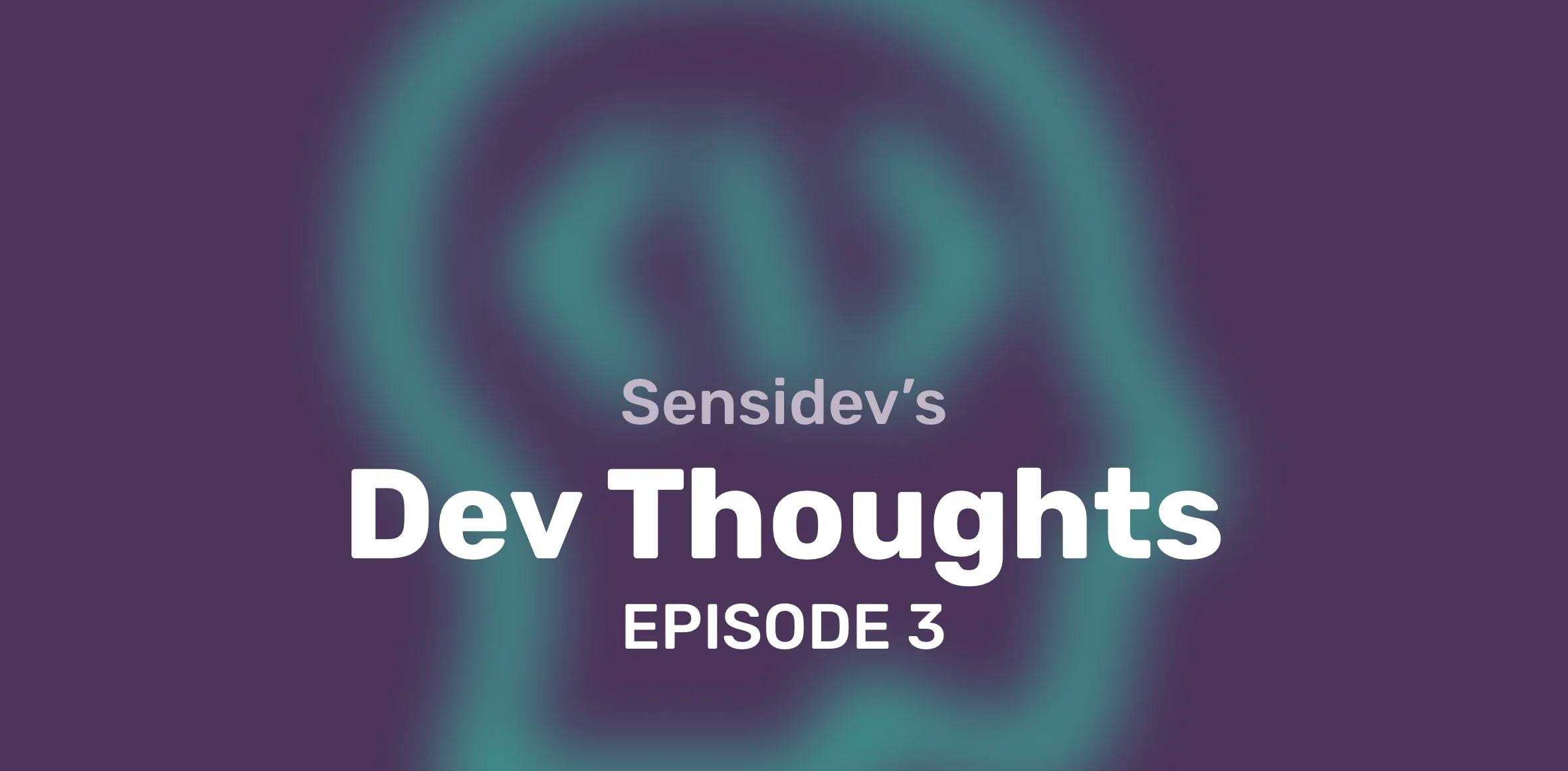
How to Create an Array with Predefined Elements in JavaScript
by Ionuț Movilă • almost 4 years ago • 3 min read
We often face the scenario of creating an array where the elements of the array depend on having an index that is incremented. How would you solve this problem?
The first attempt can look like this:
const series = new Array(3).fill({ text: '', values: [] });
Then we can just loop over the created array and add more data to it:
series.forEach((seriesValue, index) => {
seriesValue.text = `Text ${index}`;
seriesValue.values = [index, index + 1, index + 2];
});
This approach doesn't seem problematic at first until you actually run the code and the results are not at all as expected: instead of having an array with objects and the “text” prop for each object should look like: Text 0
, Text 1
and Text 2
we get objects where text prop is the same: Text 2
.
How can we explain this weird behaviour?
We’ve created an array with 3 elements that are JS objects, but what actually happened with these lines of code is that instead of defining separate objects - the fill method created a single static object, and referenced it 3 times to populate our series
array. So if we use the same object on the for each loop, this can easily explain why in the end all the array elements are equal and have the values of the last executed iteration where the index has value 2.
How can we overcome the problem?
const series = [...Array(3)].map(() => ({ text: '', values: [] }));
We create an array with 3 elements initialized to undefined, and this array will help us to create another array using the map method , that will always create a new array with distinct elements.
The second part of the code can remain the same, but in the end, when we log the results they look as expected and each object has the correct text: Text 0
, Text 1
and Text 2
and values arrays with distinct values.
Conclusion:
Using Array.fill is probably something you want to avoid because it can lead to unpredicted and hard to debug scenarios. Especially when the array is mutated over and over after being created—finding the root of the problem can be really difficult.
Dev Thoughts

How to migrate a PodBean podcast website to a custom website with Nginx permanent redirects
by Lucian Corduneanu • 4 months ago• 13 min read

The Product Owner’s View: Building a Streamlined Transport Management System
by Alexandra Voinea • 4 months ago• 5 min read

Improve Your Dev Journey: Essential Skills Beyond Just Coding
by Dragos Ispas • 5 months ago• 5 min read